Confident coding principles - Let it break (loudly) edition
Raising exceptions to define boundaries of the code can help in making sure that unwanted behavior does not go unnoticed until it is too late.
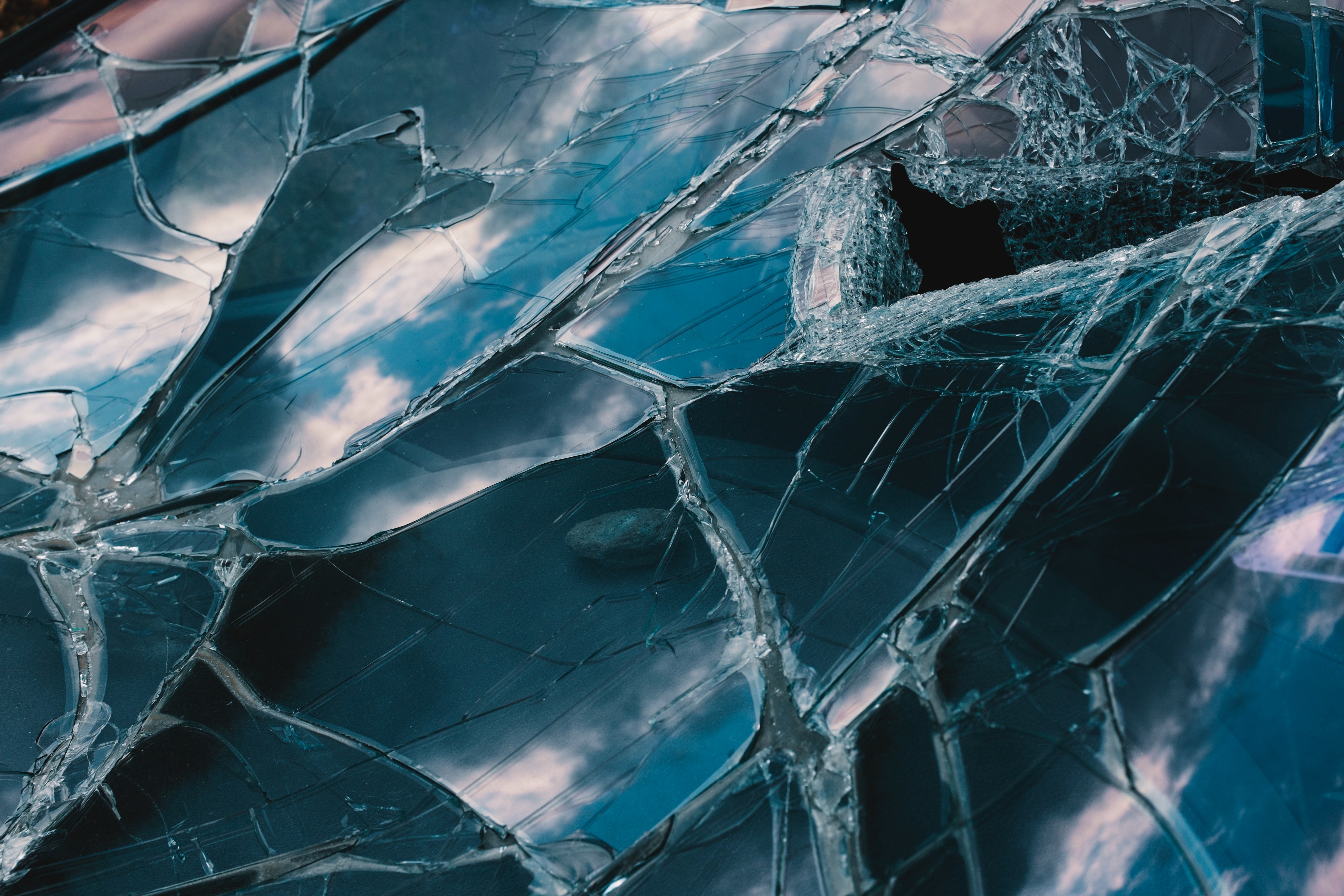
A lot of times I see code like this:
if alert_type == 'static'
do_something
else
do_something_else
end
When this code was written, we only had two types of alerts - static
and dynamic
. This code looks innocent but has a major issue. If tomorrow, we add a new alert_type
, then the code needs to change, otherwise we will process the new alert as
dynamic
alert. Worse thing is that one has to remember to change this code. Now think about such code being present at multiple places and the developer has to remember to change all the occurrences.
As programmers, we know that we are bad at remembering the code after few weeks even if it is written by ourselves. If we can forget code so quickly, surely we won't remember all the places which need to be changed.
Now this may same overkill and one can argue that is it really an issue? Well if you are making some database changes in each of those conditional blocks then your database can very well run into inconsistency. Moreover, the behavior will definitely breaks our mental model as we expected the code to work only with two alert types but now it works with other ones as well.
What if the code itself tells us that it needs to be changed? Let's try to refactor this piece of code and see if we can make the code ask us to change it and that too loudly.
if alert_type == 'static'
do_something
else if alert_type == 'dynamic'
do_something_else
else
raise "boom"
end
You might have guessed my intentions when I said loudly :) Now if we or any new developer introduces new alert type, there will be guaranteed exception which will force us to change the code OR at-least take a good look at it. This is way way better than the code silently doing wrong thing or not doing anything at all.
This post is not specific to any language, same principles can be applied in other languages apart from Ruby as well.
Raising exceptions can be good and can definitely help us in keeping the scope of the code limited and under control. If a piece of code does only one thing, it is okey as long as it doesn't do anything unexpected.
Interested in knowing more about such coding practices? Subscribe to my newsletter.